Why You Should Prefer a Single Line for C# Properties
When you write simple properties in C#, like auto properties or readonly properties that have an expression body, always put them on a single line if the code is short enough for a good one-line statement.
For example, don’t do this for an auto property:
public class Enemy
{
public bool IsDeveloper
{
get;
set;
}
}
Instead do this:
public class Friend
{
public bool IsDeveloper { get; set; }
}
Also put properties with expression bodies on a single line. For example this is great:
public class AnotherFriend
{
public bool IsDeveloper => true;
}
Don’t put your expression on a separate line like below, this is bad:
public class AnotherEnemy
{
public bool IsDeveloper
=> true;
}
What’s the advantage of a single line?
When you look at a big class, you might want to collapse it to its definitions in Visual Studio to get an overview. You can do this by using the key chord CTRL+M, CTRL+O. Alternatively you can use the main menu “Edit > Outlining > Collapse to Definitions”.
Now let’s take a look at how Visual Studio shows the collapsed enemy classes, those that don’t use a single line for their IsDeveloper property:
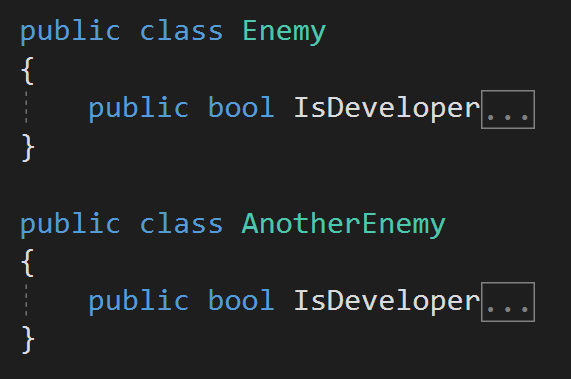
As you can see above, Visual Studio displays three dots behind the properties of the enemy classes. This is because those properties are not written on a single line. Now you have to expand such a property to see what’s inside. And it’s kind of annoying if you notice then that nothing is inside, because it’s just an auto property. It’s also annoying if the property is a readonly property that contains just a simple one-line return statement in the getter, or even has a simple one-line expression body that was just placed on a separate line (like it’s the case with the AnotherEnemy class).
Now let’s look at the collapsed friend classes that use a single line for their IsDeveloper properties. The collapsed Friend classes look like this:
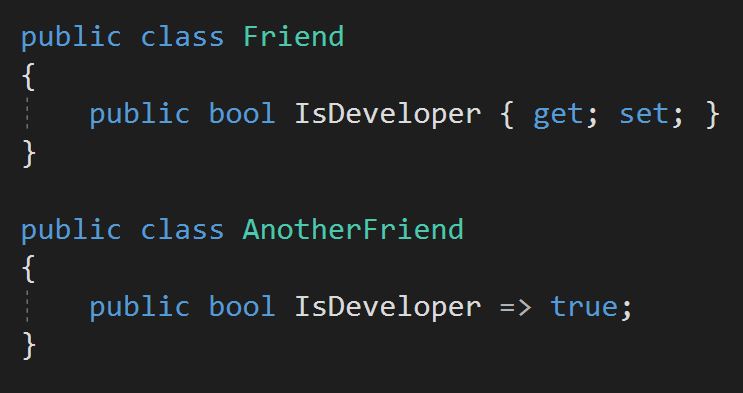
Yes, what you can see above are the collapsed friend classes. Visual Studio doesn’t display three dots if you have defined your property on a single line. Instead you can see the full-blown property. This means you don’t have to expand a property to see if it’s an auto property or just a simple readonly property with an expression body. You can see it right away without expanding the property.
So, make it easy for your friends that might want to look at your code. Write your simple properties on a single line. But of course, don’t produce lines with a character length that requires a developer to span their code editor across 4 screens to see the full line. Use multiple lines for properties that have a more complex implementation that needs multiple lines, but use a single line for auto properties and expression-bodied properties where the expression is a great fit for a single line.
Happy coding,
Thomas
Comments (2)
Can I set this rule in .editorconfig? Could not find a way to format property as you suggested automatically.
Can we add this rule to .editorconfig?